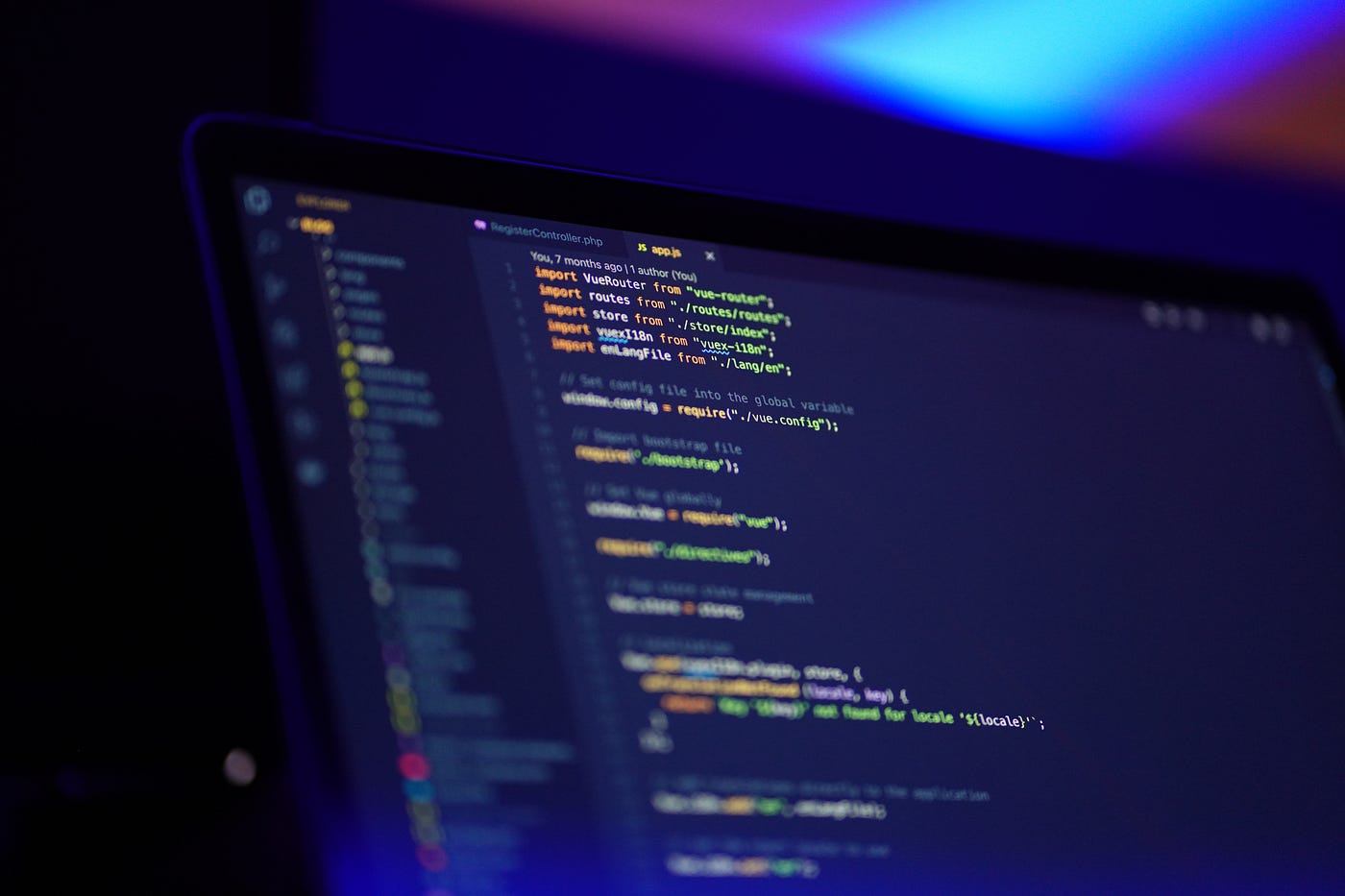
Two fundamental ideas that support object-oriented design in Java programming are inheritance and polymorphism. These guidelines encourage code reuse and abstraction, which gives developers the ability to write reliable, adaptable, and modular code. A class can inherit traits and actions from another class through inheritance, which makes the development of hierarchical class structures easier.
The ‘extends’ keyword in the syntax facilitates the creation of relationships between classes, which lays the groundwork for code management and upkeep. However, polymorphism gives objects the ability to take on many forms, which makes method implementations more flexible and dynamic method dispatch possible. Developers may construct applications that are both scalable and extendable by allowing objects to interact easily through shared interfaces.
This article explores the subtleties, syntax, and real-world uses of polymorphism and inheritance in Java, revealing the strength and beauty of these core ideas in object-oriented programming.
Inheritance: Building Hierarchies of Classes
A key idea in object-oriented programming is inheritance, which lets a new class take on the attributes and actions of an old class. This creates a relationship between the kid class (subclass) and the parent class (superclass). Reusing, extending, or overriding the parent class’s features allows the child class to encourage code reuse and abstraction.
1. Syntax of Inheritance in Java
In Java, the ‘extends’ keyword is used to implement inheritance. The syntax is simple: add “extends” to the subclass declaration, then the name of the superclass, to construct a subclass that inherits from it.
2. Types of Inheritance
Java allows for several inheritance models, such as multilayer inheritance, multiple inheritance via interfaces, and single inheritance. A class that inherits from only one superclass is said to have a single inheritance. Interfaces allow for multiple inheritance; a class can implement more than one interface. A chain of inheritance having more than two layers of classes is referred to as multilevel inheritance.
3. Access Modifiers in Inheritance
In inheritance, access modifiers (public, private, and protected) are essential. They choose which methods and fields in the subclass are visible. For instance, subclasses can access and edit a protected field in the superclass since it is visible to them.
Polymorphism: Many Forms of a Single Entity
An essential principle in Java’s object-oriented paradigm, polymorphism captures the notion of “many forms of a single entity.” The ability to express numerous implementations using a single interface or class promotes adaptability and extensibility in code. One important polymorphism mechanism is method overriding, which allows a subclass to give a particular implementation for a method that is already defined in its superclass. This adaptability makes it easier to create specialized and varied behaviours inside of a single framework.
Furthermore, polymorphism extends beyond class hierarchies to interfaces, allowing many classes to implement the same interface in their own unique ways. Java’s polymorphism, whether attained by interface implementation or method overriding, enables programmers to create modular, scalable applications that support a range of behaviours inside a single framework.
1. Method Overriding
When a subclass offers a particular implementation of a method that is already specified in its superclass, this is known as method overriding. The name, parameters, and signature of the subclass method must match those of the superclass method.
2. Interfaces and Polymorphism
In Java, interfaces are essential to creating polymorphism. Interfaces allow different classes to be treated consistently by providing a set of methods that implementing classes must follow, which promotes flexibility and code extensibility. This essential component improves Java programs’ scalability and adaptability.
3. Compile-time and Runtime Polymorphism
Runtime and compile-time polymorphism are supported by Java. Method overloading, or compile-time polymorphism, is the occurrence of two or more methods in the same class having the same name but distinct parameters. The method signature is used by the compiler to decide which method to invoke.
The Relationship Between Inheritance and Polymorphism
Effective Java object-oriented programming is built on the symbiotic relationship between inheritance and polymorphism. A subclass can inherit characteristics and actions from a superclass through inheritance, which creates a hierarchy of classes. The structure and reuse of code are based on this hierarchy. Contrarily, polymorphism presents the idea of several forms for a single entity. Because of this, objects can be viewed as instances of their parent class, which promotes extensibility and flexibility.
The way these ideas interact is demonstrated by dynamic method dispatch, in which the JVM uses an object’s runtime type to determine which method should be executed. This dynamic adaptability allows modular, maintainable, and scalable Java applications to be created, thanks to Polymorphism within an Inheritance hierarchy.
Dynamic Method Dispatch
One of the main techniques for implementing runtime polymorphism in Java is dynamic method dispatch. The Java Virtual Machine (JVM) uses the object’s actual type at runtime to determine which method should be called when one is called on it. This makes it possible to call overridden methods in the subclass with flexibility by using a reference to the superclass.
Advantages of Using Inheritance and Polymorphism
- Code Reusability: Code from pre-existing classes can be reused thanks to inheritance, which cuts down on redundancy and encourages modular code structures.
- Flexibility and Extensibility: Since new classes can be introduced to existing code without affecting it, polymorphism facilitates the construction of flexible and extendable programming.
- Improved Maintenance: Modifications to the superclass’s shared functions can be made, and through inheritance, the subclasses will immediately inherit these modifications.
- Abstraction: Because inheritance and polymorphism enable the modelling of complicated systems using more straightforward, higher-level interfaces, they aid in the abstraction process.
- Easier Debugging: Debugging is much easier when classes are arranged in a well-organized hierarchy since problems can be limited to certain classes and functions.
Best Practices and Considerations
Although polymorphism and inheritance have many advantages, they should be utilized carefully to prevent frequent problems:
- Favour Composition over Inheritance: A more adaptable option to inheritance is frequently composition, in which a class contains an instance of another class. Some of the drawbacks of strong class hierarchies are circumvented.
- Avoid Deep Class Hierarchies: Code that is difficult to maintain and complex might result from high levels of inheritance. Strive for a harmony between simplicity and reuse.
- Use Interfaces Wisely: Although interfaces are an effective technique for establishing polymorphism, using them excessively can lead to code that is extremely fragmented and difficult to understand.
- Careful with Method Overloading: Although overloading methods with unclear argument types offer flexibility, they should be avoided to avoid confusion and unexpected results.
Conclusion
In conclusion, any developer who wants to construct complex, maintainable, and scalable software must have a solid understanding of Java’s inheritance and polymorphism. The foundation of Java’s expertise in object-oriented programming is the mutually beneficial link between these two ideas. You can opt for the Java Certification Course in Gurgaon, Noida, Delhi and other parts of India.
Developers can create class hierarchies through inheritance, which promotes code reuse and clear structure. In the meantime, polymorphism adds a dynamic and adaptable layer that makes it possible for objects to take on many shapes and makes runtime flexibility easier. Through the prudent application of these concepts, developers can achieve unprecedented levels of abstraction, simplicity, and modularity in their code.
Because Java adheres to these OOP concepts, development is streamlined and software structures that are both beautiful and flexible enough to meet changing needs are created. To put it simply, knowing inheritance and polymorphism allows programmers to fully utilize Java’s capabilities and create long-lasting software.