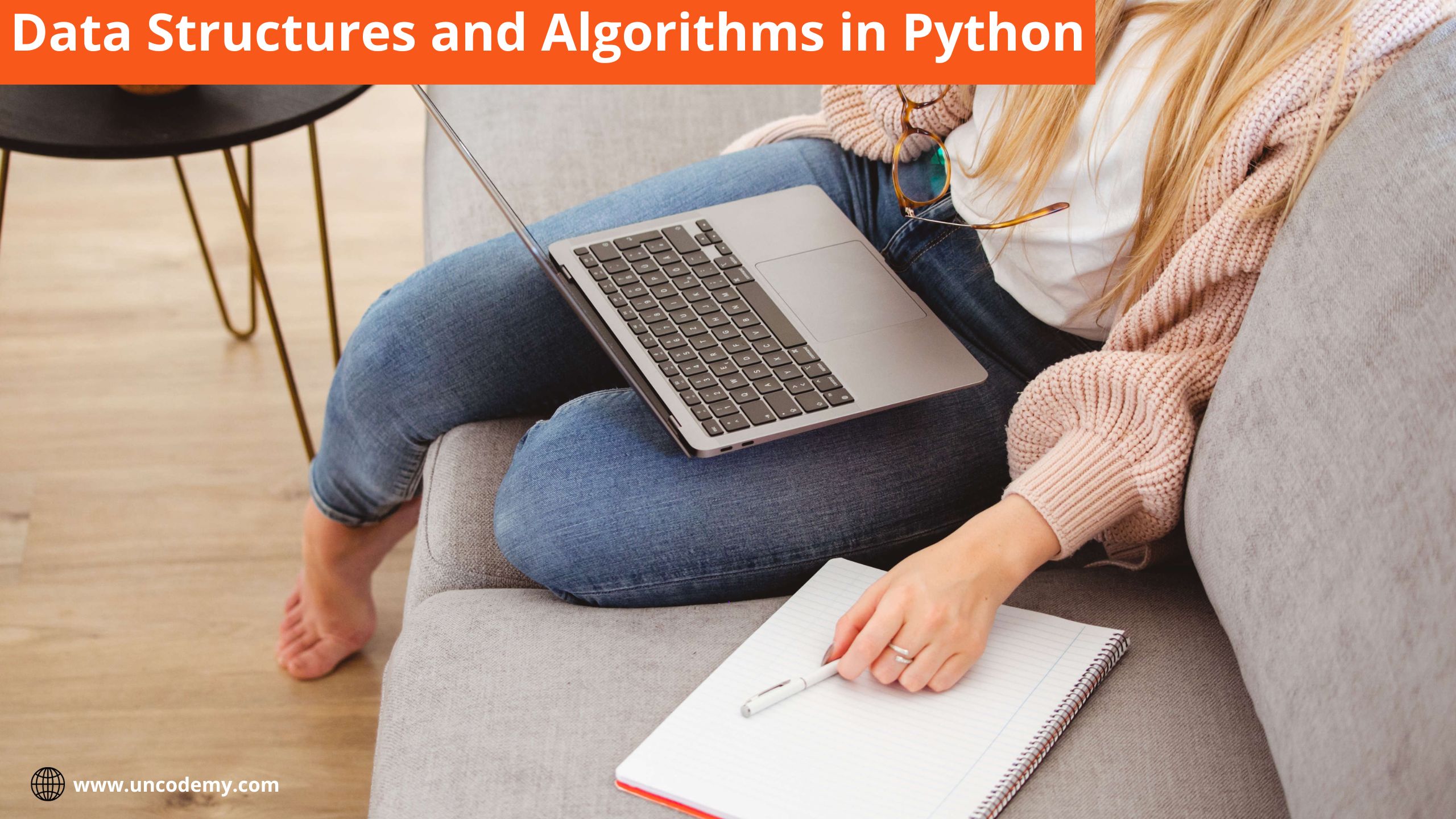
Data Structures and Algorithms in Python
Alphabets and data structures are basic ideas in computer science and programming. They are the fundamental components of software development, allowing for the effective processing, storing, and manipulating of data. We will go into the realm of data structures and algorithms in this piece, with an emphasis on Python implementations.
What Are Data Structures?
Data structures are containers for storing and organizing information. They specify how information is accessible and stored, and their effectiveness can significantly affect program performance. Python comes with a large number of built-in data structures, and you can also develop new data structures as needed.
1. Lists
One of the most utilized data structures in Python is a list. These are arranged groupings of objects, which might contain any kind of data. Lists are flexible and make it simple to manipulate data.
2. Tuples
Lists and tuples are comparable in that tuples’ elements are fixed once allocated, unlike lists. Tuples are a good option for storing data that shouldn’t be changed because of their immutability.
3. Sets
Unsorted groups with distinct components are called sets. When you need to store a group of objects without duplicates, they come in handy.
4. Dictionaries
Key-value pair groupings make up dictionaries. They offer quick and easy value lookup and retrieval based on keys.
5. Arrays
Because Python arrays hold components of the same data type, they utilize less memory than lists. To work with arrays, use the array module.
6. Stacks and Queues
Abstract data structures called stacks and queues are used to organize groups of objects with certain access patterns. The First-In, First-Out (FIFO) concept governs queues, whereas the Last-In, First-Out (LIFO) principle governs stacks.
What are Algorithms?
A collection of precise instructions for resolving a specific issue is called an algorithm. They provide step-by-step instructions on how to carry out a certain job or computation. Python is just one of the many computer languages that can be used to build algorithms, which are not dependent on language.
Common Sorting Algorithms
To arrange elements in a particular order, such as ascending or descending, sorting algorithms are utilized. There are several popular sorting algorithms that differ in their difficulty in terms of both time and space. Let’s investigate some of them.
1. Bubble Sort
A straightforward sorting method called bubble sort iteratively steps through the list, compares nearby components, and swaps them if they are out of order.
2. Selection Sort
The sorted and unsorted portions of the list are separated by selection sort. It places the minimal element at the end of the sorted portion after periodically choosing it from the unsorted portion.
3. Insertion Sort
Using an iterative process to move elements from the unsorted section into the sorted section at the appropriate places, the insert sort creates a sorted list.
Searching Algorithms
To locate a particular element in a collection, searching techniques are employed. Binary and linear search are two popular search methods.
- Linear Search
A simple technique for locating a target element in a list is linear search. Until it locates the requested item or reaches the end of the list, it goes through the list element by element.
- Binary Search
An effective search algorithm for sorted lists is binary search. Until the target element is found or the search interval is empty, it repeatedly divides the interval in half.
Time Complexity and Big O Notation
It is essential to comprehend algorithms’ time complexity in order to assess their effectiveness. Time complexity quantifies the increase in algorithm execution time as input size increases. Time complexity is often expressed using Big O notation.
Common Time Complexities
- O(1) – Constant Time: An algorithm whose execution time is constant with the size of the input. O(1) time complexity can be seen, for example, when an entry in an array is accessed using its index.
- O(log n) – Logarithmic Time: At each step, algorithms with this time complexity split the input in half. An illustration of O(log n) temporal complexity is a binary search.
- O(n) – Linear Time: Algorithms that use a linear iteration over the whole input size. An illustration of O(n) time complexity is linear search.
- O(n log n) – Linearithmic Time: Such temporal complexity is frequently found in sorting algorithms such as merge sort and quicksort.
- O(n^2) – Quadratic Time: This temporal complexity is associated with layered iterations in algorithms like bubble sort and selection sort.
- O(2^n) – Exponential Time: When the size of the input increases, algorithms with exponential time complexity do so quickly. Brutally imposed solutions to issues frequently display this kind of behavior.
- O(n!) – Factorial Time: For big inputs, algorithms with factorial time complexity should be avoided as they are quite inefficient.
Analyzing Time Complexity
We count the number of fundamental operations (such as comparisons and assignments) that an algorithm executes as a function of the input size in order to evaluate its time complexity. The growth rate of the algorithm is then expressed in terms of Big O notation using this count.
Algorithms and data structures are essential to many practical applications. Here are a few instances:
1. Web Search Engines
Sophisticated algorithms are used by search engines like Google to index content, crawl the web, and rank search results. To deliver timely and relevant search results, they use algorithms like PageRank and data structures like hash tables.
2. Databases
Data structures like hash indexes and B-trees are essential to database management systems for effective data storage and retrieval. Database queries can be expedited through the use of query optimization algorithms.
3. Games and Graphics
Data structures are used in computer graphics and game development to effectively organize geographical data. Real-time graphics and gaming depend on algorithms like collision detection and rendering improvements.
4. Machine Learning
Neural networks and decision trees are two examples of machine learning algorithms that are utilized in image recognition, natural language processing, and recommendation systems.
5. Networking
Data structures and algorithms are used by network protocols and routers to effectively route data packets over the internet. Bellman-Ford and Dijkstra routing algorithms are essential for optimal network performance.
Conclusion
To sum up, algorithms and data structures are essential parts of computer science and are essential to Python programming. Python is a great choice for developing different data structures and algorithmic solutions because of its adaptability, readability, and simplicity of usage. We have studied many different data structures along the way, including trees, graphs, stacks, queues, lists, and their accompanying search, sorting, and optimization methods. You can opt for a Python training course provider in Noida, Pune, Delhi and other parts of India.
Developers may create reliable, dependable code that can manage complicated tasks and big datasets by comprehending and using these ideas in Python. It is crucial to select the appropriate data structure and algorithm for a given problem since it has a direct impact on the scalability and performance of software programs.
Furthermore, Python’s robust third-party library and vast standard library give programmers the tools they need to take on real-world problems. Programmers can develop elegant and effective solutions that satisfy the demands of today’s quickly changing technological landscape by becoming experts in Python data structures and algorithms.