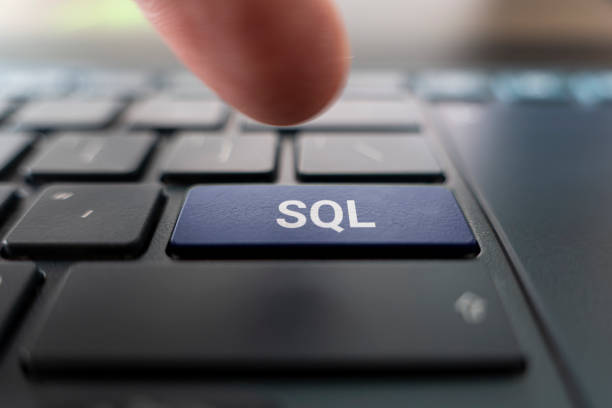
Introduction
Java, a versatile and powerful programming language, is a popular choice for software development. In Java, one of the most commonly used and essential data types is the String. Whether you’re a beginner or an experienced Java developer, mastering String manipulation is crucial for success, especially when it comes to technical interviews. In this comprehensive guide, we will delve deep into the world of Java Strings, offering valuable tips for tackling string interview questions in Java. To further enhance your preparation, we’ll also discuss Accenture previous year coding questions that frequently involve String manipulation.
Java string
Certainly! Java strings are a fundamental and widely used data type in the Java programming language. Strings in Java are objects of the `java.lang.String` class and are used to represent sequences of characters. Here’s a brief overview of Java strings:
- Immutable: One of the key characteristics of Java strings is that they are immutable, meaning their values cannot be changed once they are created. Any operation that appears to modify a string actually creates a new string object. This immutability ensures that string data remains constant, which can be advantageous for various operations.
- String Literals: In Java, you can create string objects by using string literals, which are sequences of characters enclosed in double quotes. For example:
“`java
String greeting = “Hello, World!”;
“`
- String Concatenation: Java provides various ways to concatenate strings. You can use the `+` operator, the `concat()` method, or the `StringBuilder` class for more efficient concatenation. Here’s an example using the `+` operator:
“`java
String firstName = “John”;
String lastName = “Doe”;
String fullName = firstName + ” ” + lastName;
“`
- String Methods: Java provides a wide range of methods for string manipulation. Some commonly used methods include:
- `length()`: Returns the length of the string.
- `charAt(index)`: Returns the character at the specified index.
- `substring(beginIndex, endIndex)`: Returns a substring of the original string.
- `equals(otherString)`: Compares the contents of two strings for equality.
- `indexOf(substring)`: Searches for the first occurrence of a substring in the string.
- `replace(oldChar, newChar)`: Replaces all occurrences of a character with another character.
- `split(delimiter)`: Splits the string into an array of substrings based on a delimiter.
Many more for various string manipulation tasks.
- String Comparison: When comparing strings for equality, it’s essential to use the `equals()` method, as it compares the content of the strings. Using the `==` operator for string comparison checks whether two string references point to the same memory location, not the content.
- String Pool: Java uses a string pool to store and reuse string literals. This can lead to memory optimization by reducing the memory footprint of identical strings.
- String Formatting: Java offers various methods for formatting strings, including `String.format()`, which allows you to create formatted strings using placeholders.
Tips for String Interview Questions in Java
When preparing for a Java interview, it’s essential to be well-versed in String manipulation. Interviewers often include String-related questions to evaluate a candidate’s problem-solving skills and knowledge of the language. Here are some tips to help you ace string interview questions in java:
- Understand String Basics: Start with a strong foundation. Understand what Strings are and how they are represented in Java. Strings are immutable, and they are instances of the `java.lang.String` class. Knowing this basic information will help you tackle more complex questions.
- String Methods: Be familiar with essential String methods like `length()`, `charAt()`, `substring()`, `concat()`, and `equals()`. These methods are frequently used in String manipulation and can form the basis of many interview questions.
- String Concatenation: Understand the different ways to concatenate Strings. You can use the `+` operator, the `concat()` method, or the `StringBuilder` class for efficient concatenation. Knowing when to use each method is crucial.
- String Comparison: Be aware of how to compare Strings correctly. Remember that you should use the `equals()` method for content comparison and `==` for reference comparison. Interviewers often test candidates on this distinction.
- String Searching and Manipulation: Practice String searching and manipulation techniques. Know how to find substrings using `indexOf()` and `lastIndexOf()`, and manipulate Strings with methods like `replace()` and `replaceAll()`.
- String Splitting: Learn how to split a String into an array of substrings using the `split()` method. Understanding regular expressions can be especially useful in this context.
Accenture Previous Year Coding Questions
Accenture, a global IT services company, conducts technical interviews to assess candidates for various roles, including software development. String manipulation questions are often a part of these interviews. To help you prepare, let’s take a look at some Accenture previous year coding questions that involve Strings:
- Reverse a String: Write a Java program to reverse a given String. You should provide both iterative and recursive solutions.
- String Anagrams: Create a program to check if two given Strings are anagrams of each other. Anagrams are words or phrases formed by rearranging the letters of another.
- Remove Duplicates: Write a function that removes duplicate characters from a String and returns the result. For example, “programming” should become “progamin.”
- String Compression: Implement a function that performs basic String compression. For example, “aabcccccaaa” should be compressed to “a2b1c5a3.”
- Palindrome Check: Design a program to determine if a given String is a palindrome. Ignore spaces, punctuation, and capitalization in the comparison.
- Longest Substring Without Repeating Characters: Write a function to find the length of the longest substring without repeating characters within a given String.
- String Rotation: Develop a program to check if one String is a rotation of another. For example, “waterbottle” is a rotation of “erbottlewat.”
- Count Vowels and Consonants: Create a function that counts the number of vowels and consonants in a String. Ignore spaces and consider both uppercase and lowercase characters.
Conclusion
Mastering Java Strings is a fundamental skill for any Java developer, and it’s especially important when preparing for technical interviews. By understanding String basics, familiarizing yourself with essential String methods, and practicing various String manipulation techniques, you’ll be well-equipped to tackle String-related interview questions.
In addition to these tips, reviewing Accenture previous year coding questions that involve String manipulation will provide you with practical examples of the types of challenges you might encounter in an interview. With diligent practice and a solid understanding of Strings, you can confidently approach Java interviews and increase your chances of success.
In the competitive world of software development, being proficient in Java Strings is a valuable asset. Whether you’re aiming to land your dream job at Accenture or any other tech company, this guide serves as a stepping stone to your interview success. So, prepare diligently, practice consistently, and become a master of Java Strings to reach new heights in your programming career. Good luck!